Your cart is currently empty!
NTP Clock with ESP8266 and WS2812B 8×24 Pixel LED Matrix
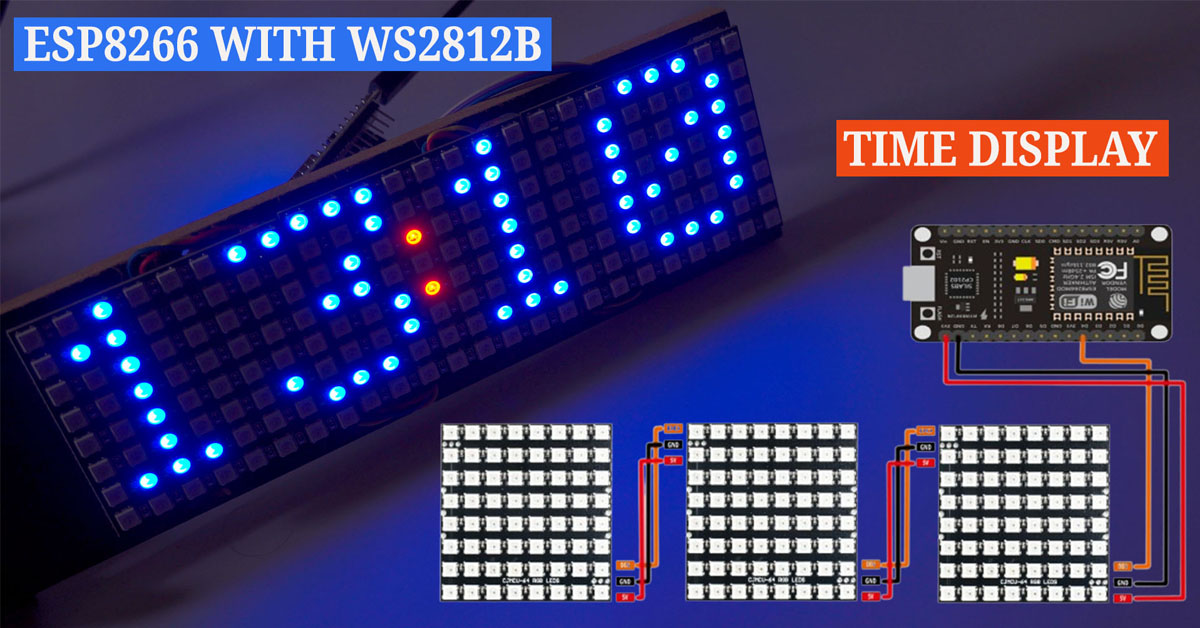
Components Used:
- Microcontroller: ESP8266
- LED Matrix: WS2812B 8×24 Pixel Matrix
- Libraries Used:
ESP8266WiFi.h
: For Wi-Fi connectivityAdafruit_GFX.h
: Core graphics library for drawing text and shapesAdafruit_NeoMatrix.h
: For controlling the NeoPixel matrixtime.h
: For handling time functions

Code Overview:
This project connects an ESP8266 microcontroller to a WS2812B 8×24 LED matrix to display the current time using the Network Time Protocol (NTP). The time is displayed in HHMM format, with a flashing effect on two vertically aligned pixels.
To connect your ESP8266 to Wi-Fi, update the ssid
and password
in the code. Look for this part:
// WiFi credentials
const char* ssid = "YourWiFiNetworkName"; // Replace with your WiFi SSID
const char* password = "YourWiFiPassword"; // Replace with your WiFi password
Replace "YourWiFiNetworkName"
with your actual Wi-Fi network name (SSID) and "YourWiFiPassword"
with your actual Wi-Fi password, keeping the quotation marks.
The time offset for Indian Standard Time (IST) based on the Greenwich Mean Time (GMT) offset.
Since IST is GMT+5:30, the offset is calculated in seconds as follows:
- 5 hours = 5 * 60 * 60 = 18,000 seconds
- 30 minutes = 30 * 60 = 1,800 seconds
- Total = 18,000 + 1,800 = 19,800 seconds
So, this offset (19,800 seconds) adjusts the time to match IST when using an NTP server.
const long gmtOffset_sec = 19800; // GMT offset for Indian Standard Time (IST) in seconds
GMT Offsets for Various Countries : click here
Key Features:
- Wi-Fi Connectivity: Connects to a specified Wi-Fi network.
- NTP Time Synchronization: Retrieves and displays the current time based on the Indian Standard Time (IST).
- Flashing Effect: Two red LEDs flash as a separator on the display.
- Customizable Brightness: The matrix brightness can be adjusted.
Code Snippet:
#include <ESP8266WiFi.h> // Include the ESP8266 WiFi library
#include <Adafruit_GFX.h> // Core graphics library
#include <Adafruit_NeoMatrix.h> // For NeoPixel Matrix
#include <time.h> // Include time library
#define DATA_PIN 2 // Use GPIO 2 for the WS2812B Data line
#define MATRIX_WIDTH 24 // Width of the matrix (24)
#define MATRIX_HEIGHT 8 // Height of the matrix (8)
#define CHAR_WIDTH 6 // Width of each character (approximation)
// WiFi credentials
const char* ssid = "YourWiFiNetworkName"; // Replace with your WiFi SSID
const char* password = "YourWiFiPassword"; // Replace with your WiFi pwd
// NTP server settings
const char* ntpServer = "pool.ntp.org"; // NTP server
const long gmtOffset_sec = 19800; // GMT offset for Indian Standard Time (IST) in seconds
const int daylightOffset_sec = 0; // No daylight saving time in India
// Initialize the matrix for a single 8x24 panel
Adafruit_NeoMatrix matrix = Adafruit_NeoMatrix(MATRIX_WIDTH, MATRIX_HEIGHT, DATA_PIN,
NEO_MATRIX_TOP + NEO_MATRIX_RIGHT + // Orientation adjustments
NEO_MATRIX_COLUMNS + NEO_MATRIX_PROGRESSIVE,
NEO_GRB + NEO_KHZ800);
// Variables for time
struct tm timeinfo;
void setup() {
// Start Serial Monitor for debugging
Serial.begin(115200);
// Initialize LED matrix
matrix.begin();
matrix.setTextWrap(false);
matrix.setBrightness(40);
matrix.setTextColor(matrix.Color(0, 0, 255)); // Set text color to blue
// Connect to Wi-Fi
Serial.print("Connecting to WiFi...");
WiFi.begin(ssid, password); // Connect to Wi-Fi
while (WiFi.status() != WL_CONNECTED) { // Wait for connection
delay(500);
Serial.print(".");
}
Serial.println(" Connected!");
// Initialize NTP
configTime(gmtOffset_sec, daylightOffset_sec, ntpServer);
}
void loop() {
// Get the current time
if (!getLocalTime(&timeinfo)) {
Serial.println("Failed to obtain time");
delay(1000);
return;
}
// Format time as HHMM
char hourString[3];
char minString[3];
snprintf(hourString, sizeof(hourString), "%02d", timeinfo.tm_hour);
snprintf(minString, sizeof(minString), "%02d", timeinfo.tm_min);
// Clear the display
matrix.fillScreen(0); // Clear the screen
// Combine hour and minute without separator
String timeString = String(hourString) + String(minString);
// Set cursor position (adjust as necessary)
matrix.setCursor(0, (MATRIX_HEIGHT - 8) / 2); // Center vertically
matrix.print(timeString); // Print the time
// Flashing effect on the 12th pixel (two pixels vertically)
static bool flash = false; // Track the flash state
if (flash) {
// Draw two vertically aligned pixels
matrix.drawPixel(11, 2, matrix.Color(255, 0, 0)); // Top pixel (one pixel up)
matrix.drawPixel(11, 4, matrix.Color(255, 0, 0)); // Bottom pixel (original position)
} else {
// Turn off the pixels
matrix.drawPixel(11, 2, matrix.Color(0, 0, 0)); // Turn off top pixel
matrix.drawPixel(11, 4, matrix.Color(0, 0, 0)); // Turn off bottom pixel
}
// Refresh the display
matrix.show();
// Toggle flash state
flash = !flash;
delay(500); // Update every half second for flashing effect
}
Usage:
- Upload the code to your ESP8266 using the Arduino IDE.
- Ensure your Wi-Fi credentials are correctly set.
- After connecting to Wi-Fi, the current time will be displayed, and the flashing effect will be visible.
Here’s a list of GMT offsets for different countries. Replace gmtOffset_sec
with the desired offset value in seconds:
const long gmtOffset_sec = <value>; // GMT offset in seconds for the chosen time zone
You can use these values to set the gmtOffset_sec
in your code based on the target country’s time zone. Remember to add or subtract based on whether the time zone is ahead or behind GMT.