Your cart is currently empty!
Measure Distance Using a Waterproof Ultrasonic Sensor with ESP32 and LCD Display
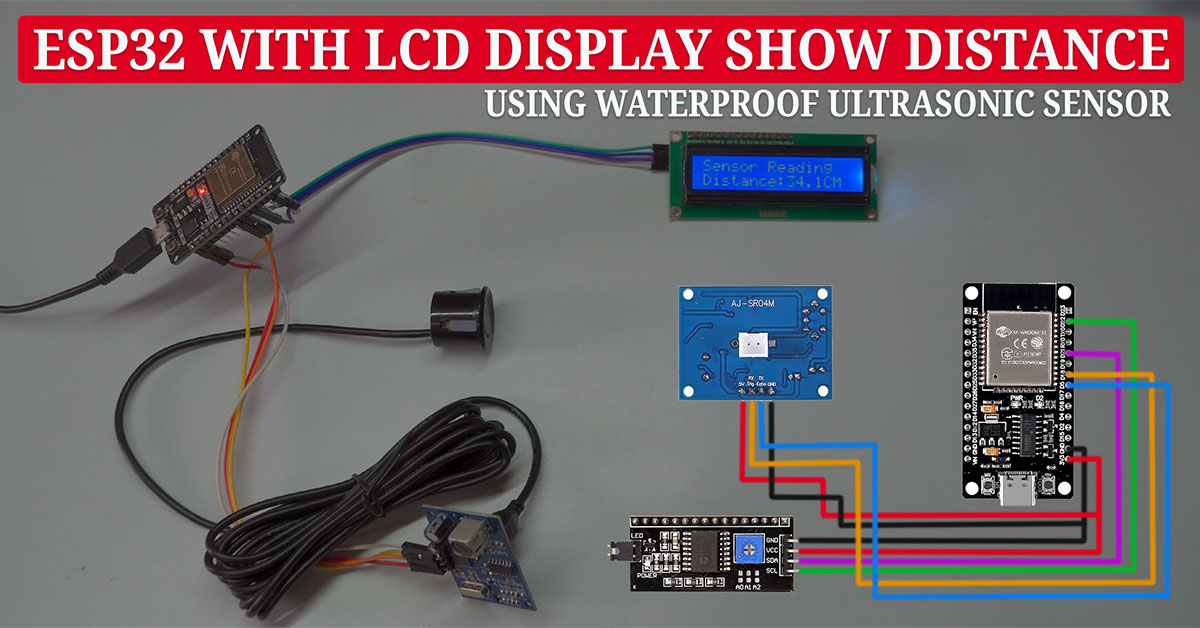
In this tutorial, we’ll guide you step-by-step on how to create a distance measurement system using an ESP32 NodeMCU, a waterproof ultrasonic sensor, and a 16×2 LCD with an I2C interface. This project is perfect for beginners and hobbyists exploring practical electronics with visual output. By the end, you’ll have a compact and functional system ideal for various applications like water level monitoring or robotics.
Components Required
To begin, gather the following components:
- ESP32 NodeMCU – the microcontroller to process data.
- I2C Interface Adapter – simplifies wiring for the LCD.
- 16×2 LCD Display – shows the real-time distance measurements.
- Waterproof Ultrasonic Sensor – measures distance accurately even in challenging environments.
Step 1: Setting up the LCD with I2C Interface
First, connect the I2C interface to the LCD. If your LCD module requires soldering, carefully align the pins before soldering them securely. A pre-soldered module with an I2C adapter can save time and reduce complexity.
The I2C interface adapter has four essential pins:
- GND connects to the ground of the ESP32.
- VCC connects to the 5V or 3.3V pin on the ESP32.
- SDA (Serial Data) links to GPIO 21 on the ESP32.
- SCL (Serial Clock) links to GPIO 22 on the ESP32.
These connections enable the ESP32 to communicate with the LCD, reducing the wiring significantly.

Step 2: Wiring the Ultrasonic Sensor
Next, wire the waterproof ultrasonic sensor to the ESP32. Connect:
- TRIG to GPIO 5 on the ESP32.
- ECHO to GPIO 18 on the ESP32.
- VCC to the 5V pin.
- GND to the ground.
This sensor will calculate the distance by emitting sound waves and measuring the time it takes for them to reflect back.

Step 3: Powering Up and Configuring
After wiring, connect the ESP32 to your PC or laptop via USB. You should see the LCD’s backlight glowing, indicating proper connection. Open the Arduino IDE, select the correct ESP32 board and COM port from the Tools menu.
Install the LiquidCrystal_PCF8574 library in the Arduino IDE for I2C LCD communication. This library allows seamless control of the LCD’s text and backlight.
Step 4: Uploading the Code
Use the provided code to calculate the distance and display it on the LCD in real time. Ensure the library is included in your code. Once uploaded, the system will measure distances and display them on the LCD.
#include <Wire.h>
#include <LiquidCrystal_PCF8574.h>
// Include sensor library if necessary
const int TRIG_PIN = 5; // Trigger pin of ultrasonic sensor
const int ECHO_PIN = 18; // Echo pin of ultrasonic sensor
LiquidCrystal_PCF8574 lcd(0x27); // LCD object with I2C address 0x27
void setup() {
Serial.begin(115200);
pinMode(TRIG_PIN, OUTPUT);
pinMode(ECHO_PIN, INPUT);
// Initialize the LCD with 16 columns and 2 rows
lcd.begin(16, 2);
lcd.setBacklight(255); // Turn on the backlight
// Display a starting message
lcd.setCursor(0, 0);
lcd.print("Ultrasonic Demo");
delay(2000);
lcd.clear();
}
void loop() {
// Trigger the ultrasonic sensor
digitalWrite(TRIG_PIN, LOW);
delayMicroseconds(2);
digitalWrite(TRIG_PIN, HIGH);
delayMicroseconds(10);
digitalWrite(TRIG_PIN, LOW);
// Measure the duration of the echo pulse
long duration = pulseIn(ECHO_PIN, HIGH);
// Calculate the distance in cm
float distance = (duration / 2.0) / 29.1;
// Display the heading on the first row
lcd.setCursor(0, 0); // Move to the first row
lcd.print("Sensor Reading"); // Heading text
lcd.print(" "); // Clear old characters, if any
// Display the distance and "CM" together on the second row
lcd.setCursor(0, 1); // Move to the second row
lcd.print("Distance:"); // Label
lcd.print(distance, 1); // Show the distance with 1 decimal
lcd.print("CM"); // Add "CM" directly after the value
lcd.print(" "); // Clear old characters, if any
delay(1000); // Wait before next reading
}


Project Features
- Compact Design: Minimal wiring using I2C simplifies the setup.
- Real-Time Display: Live updates of distance on the LCD.
- Versatile Applications: Ideal for water level monitoring, obstacle detection, parking assistance, and more.
- Scalable for IoT: The ESP32’s wireless capabilities allow future enhancements like remote monitoring.
Where Can It Be Used?
This project has numerous applications:
- Home Automation: Measure water tank levels to automate pumps.
- Robotics: Use as an obstacle detection system.
- Industrial Use: Monitor distances in assembly lines or storage units.
- Educational Tool: Great for learning about sensors, microcontrollers, and I2C communication.
This distance measurement system combines simplicity with practicality, making it a fantastic project for DIY enthu