Welcome to this step-by-step tutorial where we’ll dive into interfacing a 16×2 LCD display with an I2C interface adapter using the ESP32 Node MCU. This guide is perfect for beginners and DIY enthusiasts looking to add visual output to their electronics projects. We’ll cover everything from setting up hardware connections to writing code in the Arduino IDE to display messages on the LCD. By the end of this guide, you’ll have a solid understanding of how to connect and control an LCD display using ESP32.
Components Needed
For this project, we’ll use:
- Buy Online : ESP32 Node MCU
- Buy Online : I2C Interface Adapter
- Buy Online : 16×2 LCD Display
- Buy Online : Combo 16×2 LCD Display & I2C Interface Adapter
Let’s begin!
Step 1: Setting Up the LCD with the I2C Interface Adapter
To connect the I2C adapter to the 16×2 LCD display:
- Align the pins of the adapter with the LCD display.
- Solder the pins to secure the connection between the two components.
If you have a pre-soldered LCD with an I2C adapter, you can use that to simplify the setup and save time.
Step 2: Connecting the I2C Adapter to the ESP32
The I2C interface adapter has four main pins:
- GND: Connects to the ground on the ESP32
- VCC: Power, typically 5V (or 3.3V if your ESP32 requires it)
- SDA: Serial Data Line
- SCL: Serial Clock Line
Follow these connections:
- Connect the GND pin of the adapter to the GND on the ESP32.
- Connect the VCC pin to the 5V or 3.3V pin on the ESP32.
- Connect the SDA pin to GPIO 21 on the ESP32.
- Connect the SCL pin to GPIO 22 on the ESP32.

Once you complete the wiring, connect your ESP32 to your computer via USB. The LCD’s background LED should light up, indicating a proper connection.
In this tutorial, we demonstrated two distinct code examples for interfacing a 16×2 LCD with an I2C adapter using the ESP32. Each code plays a specific role in setting up and operating the display.
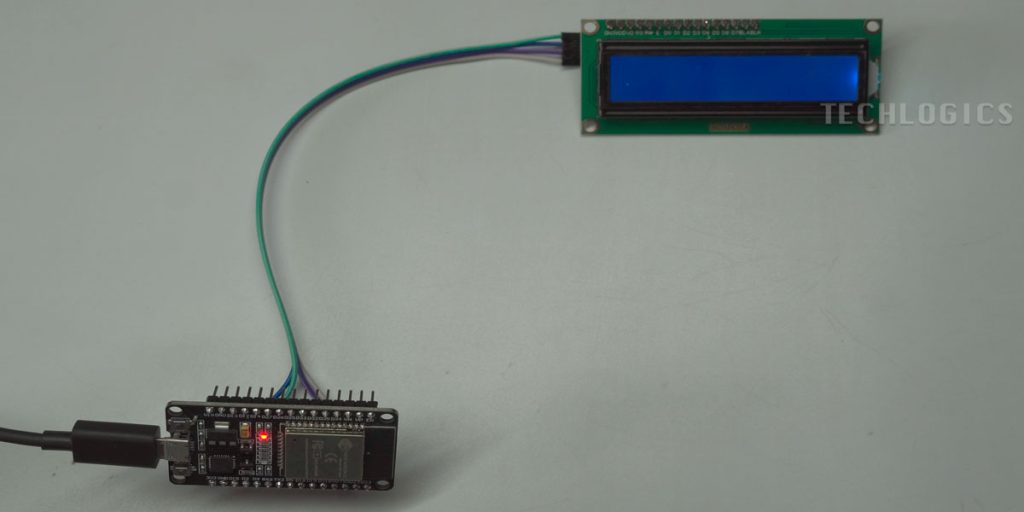
Code 1: Identifying the I2C Address of the Adapter
The first code is designed to detect the I2C address of your LCD’s adapter. Since different I2C modules may come preconfigured with various addresses (such as 0x27 or 0x3F), it’s important to identify which one your adapter uses. This step ensures that the ESP32 can communicate properly with the LCD.
- Setting Up Arduino IDE:
- Start by selecting the correct board and port in Arduino IDE. Go to Tools > Board, and choose your ESP32 model, then select the port that your ESP32 is connected to (e.g., COM3, COM4).
- Running the Code:
- Compile and upload the code to the ESP32, which will scan the I2C bus for any connected devices.
- Viewing the Address:
- After uploading, open the Serial Monitor from the Tools menu, set the baud rate to match the one specified in your code (typically 115200).
- The Serial Monitor will display the detected address, like 0x27 or 0x3F. This address confirms the I2C address of your adapter, which you will need to include in the second code.
#include <Wire.h>
void setup() {
Serial.begin(115200);
while (!Serial); // Wait for Serial to be ready (needed for some boards)
Serial.println("I2C Scanner - Scanning for devices...");
Wire.begin();
}
void loop() {
byte error, address;
int devicesFound = 0;
for (address = 1; address < 127; address++) {
Wire.beginTransmission(address);
error = Wire.endTransmission();
if (error == 0) {
Serial.print("I2C device found at address 0x");
if (address < 16)
Serial.print("0");
Serial.print(address, HEX);
Serial.println(" !");
devicesFound++;
} else if (error == 4) {
Serial.print("Unknown error at address 0x");
if (address < 16)
Serial.print("0");
Serial.println(address, HEX);
}
}
if (devicesFound == 0) {
Serial.println("No I2C devices found\n");
} else {
Serial.println("Done scanning\n");
}
delay(5000); // Wait 5 seconds before the next scan
}
This code scans all possible I2C addresses (1 to 126) to find devices connected to the I2C bus and prints the detected addresses to the Serial Monitor.
Key Steps
- Setup:
- Starts serial communication at a baud rate of 115200.
- Initializes I2C communication using
Wire.begin()
.
- Loop:
- Checks each I2C address (1–126) to see if a device responds.
- If a device is found, it prints the address to the Serial Monitor.
- If there’s an error at any address, it reports it as an unknown error.
- Result:
- If no devices are found, it displays “No I2C devices found.”
- Otherwise, it prints “Done scanning” and repeats the scan every 5 seconds.
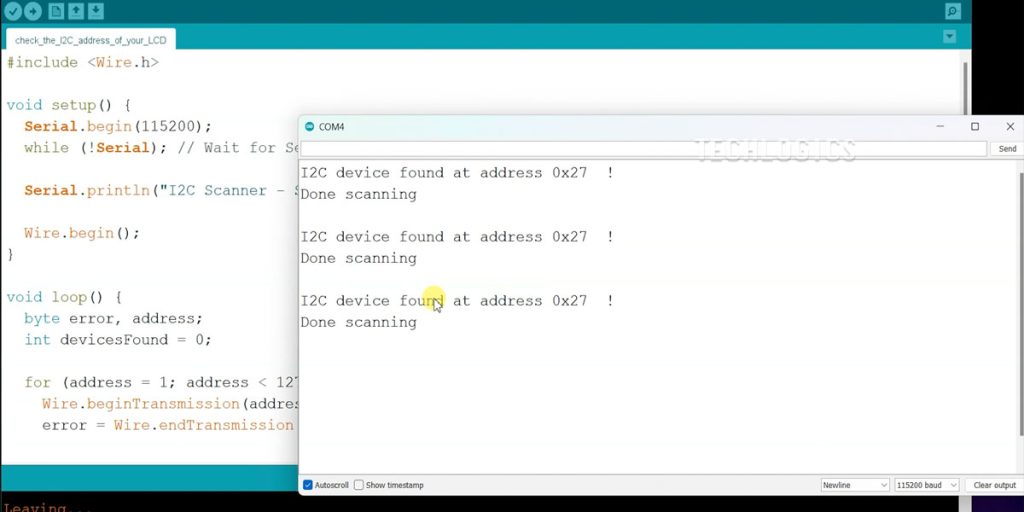
Code 2: Displaying Text on the LCD
Once you have the I2C address, the second code lets you display custom text on the LCD.
- Installing the Required Library:
- To work with the LCD, you’ll need to install the
LiquidCrystal_PCF8574
library, which provides the necessary functions to control the display. - In Arduino IDE, go to Sketch > Include Library > Manage Libraries, then search for
LiquidCrystal_PCF8574
and install it.
- To work with the LCD, you’ll need to install the
- Modifying the Code:
- Open the code for displaying text on the LCD. In this code, update the I2C address to the one you found earlier (e.g.,
0x27
). This ensures that the ESP32 communicates accurately with your specific adapter.
- Open the code for displaying text on the LCD. In this code, update the I2C address to the one you found earlier (e.g.,
- Uploading the Code:
- Compile and upload the modified code to your ESP32. After uploading, the text you specified in the code should appear on the LCD screen.
#include <Wire.h>
#include <LiquidCrystal_PCF8574.h>
// Create an LCD object using the detected I2C address 0x27
LiquidCrystal_PCF8574 lcd(0x27);
void setup() {
Serial.begin(115200);
// Initialize the LCD with 16 columns and 2 rows
lcd.begin(16, 2);
// Turn on the backlight
lcd.setBacklight(255);
}
void loop() {
// Display a new message on the LCD
lcd.setCursor(0, 0); // Set cursor to the first line
lcd.print("Testing LCD...");
lcd.setCursor(0, 1); // Set cursor to the second line
lcd.print("Address: 0x27");
// Keep the message on screen for 2 seconds
delay(2000);
// Clear the LCD screen
lcd.clear();
// Display a second message on the LCD
lcd.setCursor(0, 0);
lcd.print("I2C Communication");
lcd.setCursor(0, 1);
lcd.print("Successful!");
// Keep the second message on screen for 2 seconds
delay(2000);
// Clear the screen before starting over
lcd.clear();
}
This code displays messages on a 16×2 LCD screen using an I2C interface at address 0x27
.
Key Components
- Libraries:
Wire.h
andLiquidCrystal_PCF8574.h
enable communication with the I2C-based LCD.
- LCD Initialization:
- An LCD object is created using the address
0x27
. - The LCD screen is set up with 16 columns and 2 rows, and the backlight is turned on.
- An LCD object is created using the address
- Display Messages in the Loop:
- First Message:
- Displays “Testing LCD…” on the first line.
- Displays “Address: 0x27” on the second line.
- Keeps this message visible for 2 seconds.
- Second Message:
- Clears the screen, then shows “I2C Communication” on the first line.
- Shows “Successful!” on the second line.
- Keeps this message visible for 2 seconds.
- First Message:
- Loop Repeat:
- After displaying both messages, the screen clears, and the loop repeats, continually displaying the messages with pauses in between.
Adjusting LCD Display Settings
If the text on the LCD appears faint or unclear, you may need to adjust the potentiometer on the back of the I2C adapter. This small knob controls the display contrast, allowing you to adjust brightness and make the text easier to read.
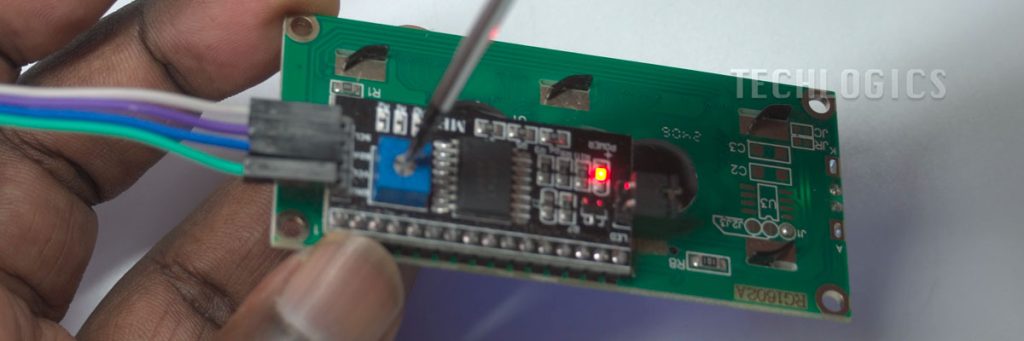
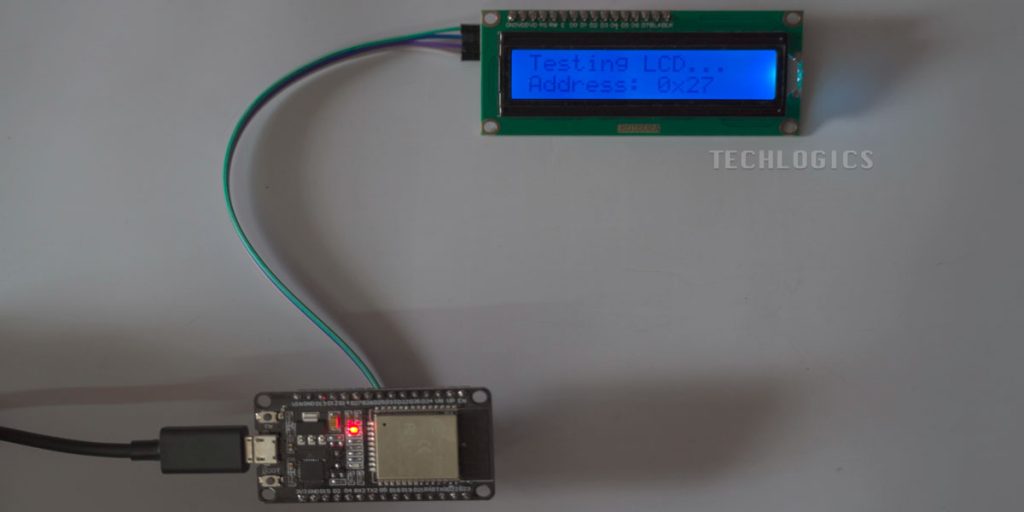
Troubleshooting and Additional Resources
If your LCD doesn’t display text after following these steps:
- Double-check your wiring between the ESP32 and I2C adapter.
- Confirm the I2C address using the first code if you encounter communication errors.
For more detailed explanations, additional code examples, and troubleshooting tips, you can refer to other videos in this series, where we dive deeper into various LCD projects.