Your cart is currently empty!
Display YouTube Subscriber Count on MAX7219 8-Digit 7-Segment Display Using ESP32 Node MCU | Wiring & Code Tutorial
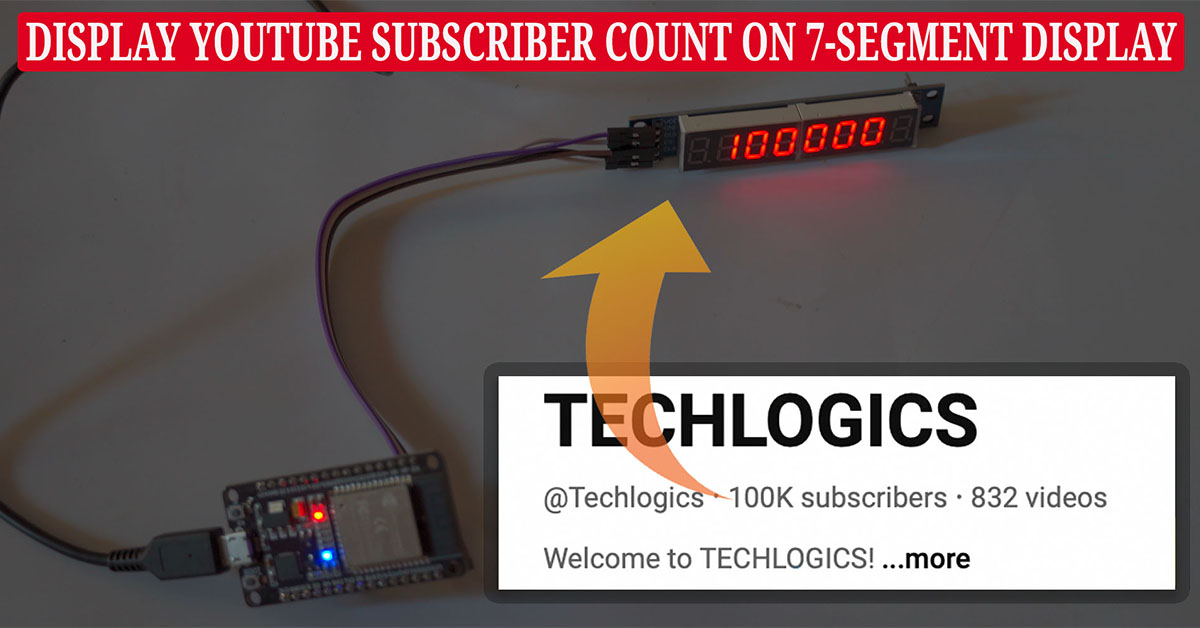
In this tutorial, we will walk you through the process of displaying your YouTube subscriber count on a MAX7219 8-Digit 7-Segment Display using an ESP32 Node MCU. Whether you’re a YouTuber looking to showcase live stats or an electronics enthusiast diving into IoT projects, this step-by-step guide is for you!
Step 1: Materials Required
Before starting the wiring and coding, ensure you have the following materials ready:
- ESP32 Node MCU
- MAX7219 8-Digit 7-Segment Display
- Jumper wires
- Breadboard (optional)
- USB cable to connect ESP32 to your PC
Step 2: Wiring the MAX7219 to ESP32
Start by connecting the MAX7219 8-Digit 7-Segment Display to the ESP32 Node MCU. Follow the detailed wiring setup:
- Power Connections:
- 3.3V (ESP32) → VCC (MAX7219)
- GND (ESP32) → GND (MAX7219)
- Data Connections:
- DIN (MAX7219) → GPIO 23 (ESP32) (Data input pin for controlling the display)
- CLK (MAX7219) → GPIO 18 (ESP32) (Clock pin to synchronize data timing)
- CS (MAX7219) → GPIO 5 (ESP32) (Chip select pin to enable display)
This wiring will ensure that the ESP32 can communicate with the MAX7219 display to show the subscriber count.

Buy Online : ESP32 Node MCU

Buy Online : max7219 8-digit display

Step 3: Powering Up
After the wiring is complete, connect your ESP32 Node MCU to your PC using a USB cable. This powers both the ESP32 and the MAX7219 display module.
Step 4: Setting Up Arduino IDE for ESP32
- Install the ESP32 Board:
- Open the Arduino IDE on your PC.
- Go to File > Preferences.
- In the Additional Boards Manager URLs section, add this URL:
https://dl.espressif.com/dl/package_esp32_index.json
. - Now, go to Tools > Board > Board Manager and search for ESP32. Click Install for the ESP32 by Espressif.
- Select Your ESP32 Board:
- Go to Tools > Board and select ESP32 Dev Module (or the exact ESP32 model you’re using).
- Choose the Port corresponding to your ESP32 under Tools > Port.
Step 5: Obtain YouTube API Key
To fetch your YouTube subscriber count, you’ll need a YouTube API key. Here’s how you can obtain one:
- Go to Google Cloud Console:
- Open the Google Cloud Console.
- Sign in with your Google account linked to your YouTube channel.
- Create a New Project:
- Click on the Select a Project dropdown at the top and choose New Project.
- Name your project (e.g., “YouTube Subscriber Counter”) and click Create.
- Enable YouTube Data API:
- Go to API & Services > Dashboard and click on Enable APIs and Services.
- In the search bar, type YouTube Data API v3 and enable it for your project.
- Generate API Key:
- In the left-hand sidebar, go to Credentials.
- Click on Create Credentials and choose API Key.
- A wizard will open, guiding you through the process. From the options provided, select Public Data, as this project only requires access to publicly available YouTube subscriber information. After selecting Public Data, click Next, and then choose API Key as the credential type.
- Copy the generated API Key. This key is required for the ESP32 to access your channel’s data.










Step 6: Get Your YouTube Channel ID
Your Channel ID is required to fetch the subscriber count. Here’s how to find it:
- Using YouTube Studio:
- Open YouTube Studio.
- Click on Settings in the left sidebar.
- Navigate to Advanced Settings, and you’ll find the Channel ID listed there.
- Using YouTube Website:
- Open your YouTube Channel in a browser.
- Look at the URL, which should look like:
https://www.youtube.com/channel/UC1234567890abcdef
. - The string afterÂ
/channel/
 is your Channel ID.

Step 7: Writing the Arduino Code
- Install the Required Library:
- To control the MAX7219 display, install the LedControl library:
- Go to Sketch > Include Library > Manage Libraries.
- Search for LedControl and click Install.
- To control the MAX7219 display, install the LedControl library:
#include <WiFi.h>
#include <LedControl.h>
#include <HTTPClient.h>
#include <ArduinoJson.h>
// WiFi credentials
const char* ssid = "YOUR_WIFI_SSID";
const char* password = "YOUR_WIFI_PASSWORD";
// YouTube API key and channel ID
const char* apiKey = "YOUR_API_KEY"; // Replace with your YouTube Data API key
const char* channelId = "YOUR_CHANNEL_ID"; // Replace with your YouTube channel ID
// Create a LedControl instance (DIN, CLK, CS, number of MAX7219 chips)
LedControl lc = LedControl(23, 18, 5, 1); // DIN to GPIO 23, CLK to GPIO 18, CS to GPIO 5
void setup() {
Serial.begin(115200);
// Connect to WiFi
Serial.println("Connecting to WiFi...");
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.print(".");
}
Serial.println("\nConnected to WiFi");
Serial.print("IP Address: ");
Serial.println(WiFi.localIP());
// Initialize the MAX7219
lc.shutdown(0, false); // Wake up the MAX7219
lc.setIntensity(0, 5); // Set brightness level (0-15)
lc.clearDisplay(0); // Clear display register
// Test the display with a sample number
// String testNumber = "87654321"; // Replace with any test number
Serial.println("Displaying test number...");
// displayTestNumber(testNumber); // Test the display
}
void loop() {
// Fetch and display the subscriber count every 60 seconds
String subscriberCount = getSubscriberCount();
if (subscriberCount != "0") { // Only update if valid data is retrieved
Serial.print("YouTube Subscriber Count: ");
Serial.println(subscriberCount);
displaySubscriberCount(subscriberCount);
}
delay(60000); // Wait for 60 seconds before updating again
}
// Function to fetch the subscriber count from YouTube API
String getSubscriberCount() {
HTTPClient http;
String url = "https://www.googleapis.com/youtube/v3/channels?part=statistics&id=" + String(channelId) + "&key=" + String(apiKey);
http.begin(url);
int httpCode = http.GET();
String subscriberCount = "0"; // Default value in case of error
if (httpCode == 200) {
String payload = http.getString();
DynamicJsonDocument doc(1024);
deserializeJson(doc, payload);
subscriberCount = doc["items"][0]["statistics"]["subscriberCount"].as<String>();
} else {
Serial.println("Error fetching data");
}
http.end();
return subscriberCount;
}
// Function to display the subscriber count on the 7-segment display
void displaySubscriberCount(String subscriberCount) {
lc.clearDisplay(0); // Clear the display first
int displayLength = 8; // Total digits on the display
int countLength = subscriberCount.length(); // Length of the subscriber count
int startPos = (displayLength - countLength) / 2; // Calculate the starting position for centering
// Loop through the subscriber count and display each digit in the correct order
for (int i = 0; i < displayLength; i++) {
if (i >= startPos && i < startPos + countLength) {
char c = subscriberCount.charAt(i - startPos); // Display in normal order
if (c >= '0' && c <= '9') {
lc.setDigit(0, displayLength - 1 - i, c - '0', false); // Adjust for 7-segment positioning
} else {
lc.setChar(0, displayLength - 1 - i, ' ', false); // Display a space for non-numeric characters
}
} else {
lc.setChar(0, displayLength - 1 - i, ' ', false); // Fill the rest with spaces
}
}
}
// Function to display a test number on the 7-segment display
void displayTestNumber(String testNumber) {
lc.clearDisplay(0); // Clear the display first
int displayLength = 8; // Total digits on the display
int countLength = testNumber.length(); // Length of the test number
int startPos = (displayLength - countLength) / 2; // Calculate the starting position for centering
// Loop through the test number and display each digit in the correct order
for (int i = 0; i < displayLength; i++) {
if (i >= startPos && i < startPos + countLength) {
char c = testNumber.charAt(i - startPos); // Display in normal order
if (c >= '0' && c <= '9') {
lc.setDigit(0, displayLength - 1 - i, c - '0', false); // Adjust for 7-segment positioning
} else {
lc.setChar(0, displayLength - 1 - i, ' ', false); // Display a space for non-numeric characters
}
} else {
lc.setChar(0, displayLength - 1 - i, ' ', false); // Fill the rest with spaces
}
}
}
- Edit the Code:
- Open the Arduino IDE and paste the provided code for retrieving the YouTube subscriber count.Update the following lines in the code:
- WiFi Credentials: ReplaceÂ
ssid
 andÂpassword
 with your WiFi network details.API Key: ReplaceÂ"YOUR_API_KEY"
 with the API key you obtained earlier.Channel ID: ReplaceÂ"YOUR_CHANNEL_ID"
 with your YouTube channel ID.
- WiFi Credentials: ReplaceÂ
- Open the Arduino IDE and paste the provided code for retrieving the YouTube subscriber count.Update the following lines in the code:
const char* ssid = "YOUR_WIFI_SSID";
const char* password = "YOUR_WIFI_PASSWORD";
const String apiKey = "YOUR_API_KEY";
const String channelID = "YOUR_CHANNEL_ID";
- Verify and Upload:
- After adding your credentials and API key, click the Verify button to check for errors in the code.
- Once verified, click Upload to transfer the code to your ESP32.
Step 8: Testing the Setup
Once the code has successfully uploaded to the ESP32, the MAX7219 display will show your YouTube subscriber count. Additionally, the serial monitor will output:
- WiFi connection status (connected or failed).
- Real-time subscriber count retrieved from the YouTube API.


Step 9: Enjoy Your Live YouTube Subscriber Display!
With everything set up and working, your MAX7219 display should now continuously update with your YouTube subscriber count in real-time.
If this tutorial helped you, please like, share, and subscribe to support us! Your feedback makes a huge difference and encourages us to create more content like this.
test
For ESP8266 code available?