Your cart is currently empty!
Display Numbers on MAX7219 8-Digit 7 Segment Display Using ESP32 Node MCU | Wiring & Code Tutorial
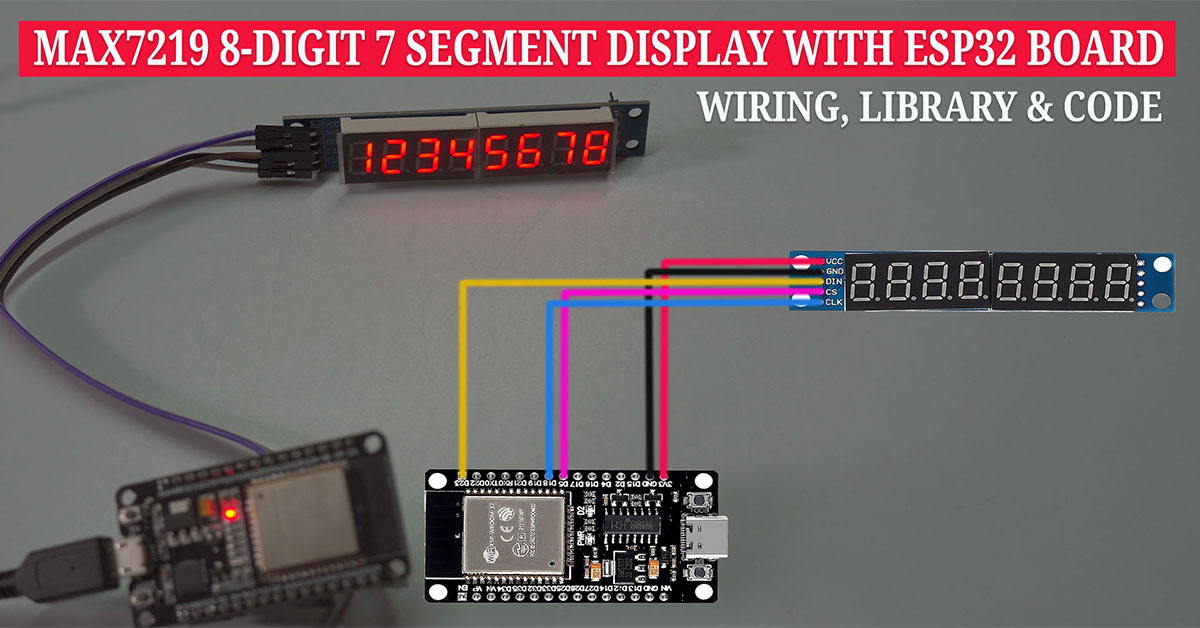
Introduction
In this tutorial, we’ll show you how to wire and code a MAX7219 8-Digit 7 Segment Display with an ESP32 Node MCU to display numbers. This project is beginner-friendly and great for DIY enthusiasts who want to add a simple visual output to their electronics projects. By following along, you’ll learn how to set up hardware connections, install libraries in the Arduino IDE, and write code that sends numbers to the display for easy control and customization.
Components Needed
Step 1: Wiring the ESP32 to the MAX7219 Display
To set up the connections, start by linking the power pins. Here’s a quick overview:
- Power Connections:
- Connect the 3.3V pin on the ESP32 to the VCC pin on the MAX7219.
- Connect GND on the ESP32 to GND on the MAX7219.
- Data Connections:
- Connect DIN on the MAX7219 to GPIO 23 on the ESP32 for data input.
- Connect CLK on the MAX7219 to GPIO 18 for data timing.
- Connect CS on the MAX7219 to GPIO 5 on the ESP32 for module selection.
Once wired, connect your ESP32 to your PC or laptop via a USB cable. This will power both the ESP32 and the MAX7219 display.


Step 2: Setting Up Arduino IDE and Installing Libraries
Installing the LedControl Library
To control the MAX7219 display with the ESP32 in the Arduino IDE, we need the LedControl library:
- Open the Arduino IDE and go to Sketch > Include Library > Manage Libraries.
- In the Library Manager, search for LedControl and install it (it’s by Eberhard Fahle).
- Confirm it’s installed by checking under Sketch > Include Library.

Configuring the IDE
To ensure smooth communication between your ESP32 and the MAX7219:
- Go to Tools > Board and select the appropriate ESP32 board.
- Under Port, choose the correct port for your ESP32.
Step 3: Writing and Uploading Code
With the library ready, it’s time to upload a basic code to display numbers on the MAX7219:
#include <LedControl.h>
// Create a LedControl instance (DIN, CLK, CS, number of MAX7219 chips)
LedControl lc = LedControl(23, 18, 5, 1); // DIN to GPIO 23, CLK to GPIO 18, CS to GPIO 5
// Function declaration
void displayNumber(long num);
void setup() {
// Initialize the MAX7219
lc.shutdown(0, false); // Wake up the MAX7219
lc.setIntensity(0, 8); // Set brightness level (0-15)
lc.clearDisplay(0); // Clear display register
}
void loop() {
displayNumber(12345678); // Display the number
delay(2000); // Wait for 2 seconds
}
// Function definition
void displayNumber(long num) {
// Display each digit on the display
for (int i = 0; i < 8; i++) {
int digit = num % 10; // Get the last digit of num
lc.setDigit(0, i, digit, false); // Set the digit on the display
num /= 10; // Remove the last digit from num
}
}
This code displays 12345678
on the MAX7219 display. To customize, replace 12345678
with any other number you wish to display.
Step 4: Troubleshooting and Common Errors
If you encounter a fatal error: avr/pgmspace.h during compilation:
- Open the LedControl library folder (typically in Arduino/libraries).
- In LedControl.cpp or LedControl.h, find the line
#include <avr/pgmspace.h>
and comment it out (add//
at the beginning). - Save the file and try compiling again.


Step 5: Uploading Code to the ESP32
Once your code compiles without errors:
- Connect the ESP32 to your computer via USB.
- In the Arduino IDE, go to Tools > Board and Tools > Port to select the correct settings.
- Click the Upload button.
Once the upload completes, the ESP32 will run the code, and you should see the number appear on the MAX7219 display.
Conclusion
In this tutorial, you learned how to set up a MAX7219 8-Digit 7 Segment Display with an ESP32 Node MCU, install libraries, and upload code to display numbers. This setup opens the door to adding more visual elements to your projects, and we hope it inspires you to explore even more with LED displays!