Your cart is currently empty!
Create an Eye-Catching Scrolling and Flashing Text Display with ESP8266 and WS2812B LED Panel
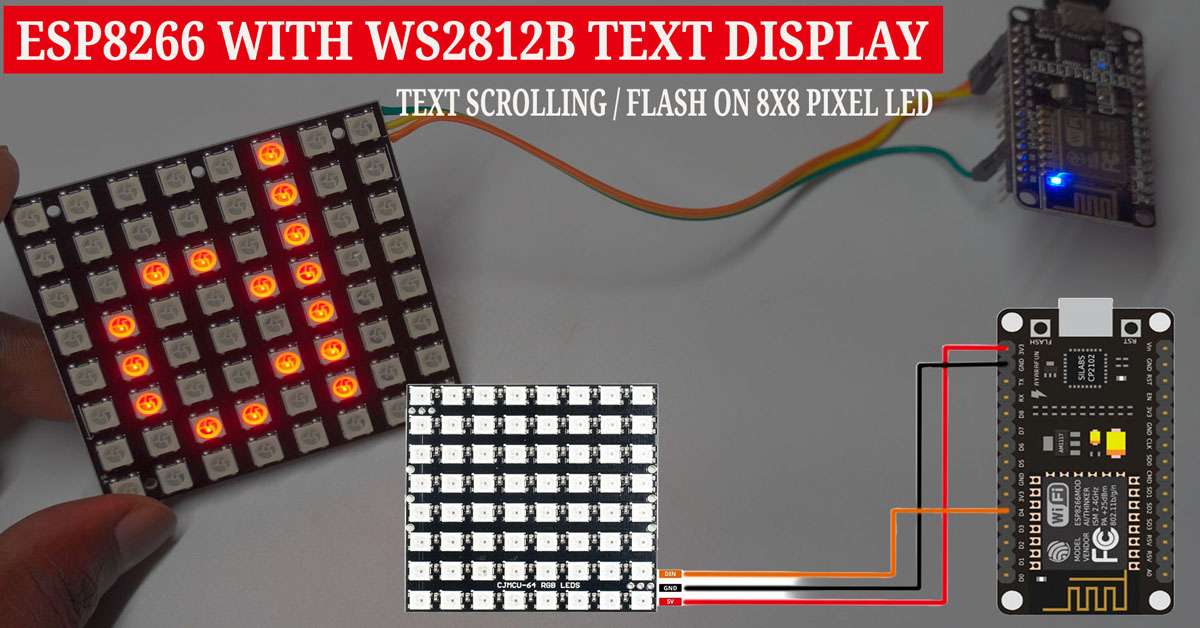
In this tutorial, we’ll walk you through creating a vibrant and dynamic scrolling and flashing text display using an ESP8266 board and a WS2812B 8×8 LED panel. This project is perfect for enhancing your DIY electronics setup, allowing you to add a colorful, programmable LED display to your projects.
Components Needed
To get started, you’ll need a few essential components:
- ESP8266 Board: This will be your primary controller, managing the animations and sending commands to the LEDs. You can use boards like the NodeMCU or Wemos D1 Mini.
- WS2812B 8×8 LED Matrix Panel: This panel will display the colorful scrolling and flashing text, with each LED individually addressable for various effects.
- 5V Power Supply: A stable 5V power supply is crucial, as the LED panel requires more current than the ESP8266 alone can provide.
- Jumper Wires: You’ll need these to connect the ESP8266’s data, power, and ground connections to the LED matrix.
Buy ESP8266 Board Online : https://amzn.to/4houB69
Buy WS2812B 8×8 LED Online : https://amzn.to/3AiSsng
Hardware Setup
- Prepare the LED Matrix: Start by soldering jumper wires to the power, ground, and data input pins on the WS2812B 8×8 LED Matrix Panel to ensure reliable connections. Identify the +5V, GND, and Data In (DIN) pins on the LED matrix panel, then solder one jumper wire to each of these pins.
- Connect the Wires:
- Connect the 5V jumper wire from the LED matrix to an external 5V power supply (preferably 5V 2A or more).
- Connect the GND wire from the LED matrix to the GND pin on the ESP8266, as well as the GND terminalof the external power supply to establish a common ground.
- Finally, attach the DIN jumper wire from the LED matrix to a digital GPIO pin on the ESP8266, typically D4 as specified in the code.
Note: While this demonstration uses the board’s power supply to power the LED panel at minimum brightness, an external DC 5V power source is recommended for optimal functionality.

Software Setup
Uploading the Code
- Open Arduino IDE: Ensure your ESP8266 is connected to your computer via USB.
- Select Board and Port: In the Arduino IDE, select the appropriate ESP board from the board manager and choose the correct Arduino port for your device.
- Install Required Libraries: You need to install the Adafruit GFX Library for graphics capabilities and the Adafruit NeoPixel Library for controlling WS2812B LEDs. Go to
Sketch > Include Library > Manage Libraries
, search for each library, and click “Install”.
Code for Scrolling Text
Here’s the code to display scrolling text in red:
#include <Adafruit_GFX.h> // Core graphics library
#include <Adafruit_NeoMatrix.h> // For NeoPixel Matrix
#include <Adafruit_NeoPixel.h> // Basic NeoPixel library
#define DATA_PIN 2 // Use GPIO 2 for the WS2812B Data line
#define MATRIX_WIDTH 8 // Width of the matrix (8x8)
#define MATRIX_HEIGHT 8 // Height of the matrix
// Initialize the matrix with different orientation
Adafruit_NeoMatrix matrix = Adafruit_NeoMatrix(MATRIX_WIDTH, MATRIX_HEIGHT, DATA_PIN,
NEO_MATRIX_TOP + NEO_MATRIX_RIGHT + // Change NEO_MATRIX_LEFT to NEO_MATRIX_RIGHT
NEO_MATRIX_COLUMNS + NEO_MATRIX_PROGRESSIVE,
NEO_GRB + NEO_KHZ800);
// Your scrolling message
char message[] = "Hello, World!";
int textPos = MATRIX_WIDTH; // Start the text off-screen to the right
void setup() {
matrix.begin();
matrix.setTextWrap(false); // Disable text wrapping
matrix.setBrightness(40); // Set brightness (0-255)
matrix.setTextColor(matrix.Color(255, 0, 0)); // Set text color (Red)
}
void loop() {
matrix.fillScreen(0); // Clear the screen
matrix.setCursor(textPos, 0); // Position the text
matrix.print(message); // Print the message
matrix.show(); // Refresh the display
delay(100); // Delay for scrolling speed
// Move the text left
textPos--;
// Reset the position when the text has fully scrolled off the left
int16_t textWidth = 6 * strlen(message); // 6 pixels per character for size 1
if (textPos < -textWidth) {
textPos = MATRIX_WIDTH; // Reset position to scroll again
}
}
Customizing Your Display
To display your own text, simply replace the text in the message
variable. For example, change the line:
char message[] = "Hello, World!";
to
char message[] = "Your Text Here!";
Ensure to keep the quotation marks around your new text. After modifying the code, upload it to your ESP8266, and your customized message will display on the LED matrix.
You can adjust the brightness and text color using these functions:
- Set Brightness:
matrix.setBrightness(value);
(0-255) - Set Text Color:
matrix.setTextColor(matrix.Color(r, g, b));
(adjust RGB values)

Colorful Scrolling Text
Now, let’s enhance the visual appeal by changing each letter’s color as it scrolls. Here’s the code:
#include <Adafruit_GFX.h> // Core graphics library
#include <Adafruit_NeoMatrix.h> // For NeoPixel Matrix
#include <Adafruit_NeoPixel.h> // Basic NeoPixel library
#define DATA_PIN 2 // Use GPIO 2 for the WS2812B Data line
#define MATRIX_WIDTH 8 // Width of the matrix (8x8)
#define MATRIX_HEIGHT 8 // Height of the matrix
// Initialize the matrix with different orientation
Adafruit_NeoMatrix matrix = Adafruit_NeoMatrix(MATRIX_WIDTH, MATRIX_HEIGHT, DATA_PIN,
NEO_MATRIX_TOP + NEO_MATRIX_RIGHT + // Change NEO_MATRIX_LEFT to NEO_MATRIX_RIGHT
NEO_MATRIX_COLUMNS + NEO_MATRIX_PROGRESSIVE,
NEO_GRB + NEO_KHZ800);
// Your scrolling message
char message[] = "Hello, World!";
int textPos = MATRIX_WIDTH; // Start the text off-screen to the right
// Define some colors
uint16_t colors[] = {
matrix.Color(255, 0, 0), // Red
matrix.Color(0, 255, 0), // Green
matrix.Color(0, 0, 255), // Blue
matrix.Color(255, 255, 0), // Yellow
matrix.Color(255, 0, 255), // Magenta
matrix.Color(0, 255, 255), // Cyan
matrix.Color(255, 165, 0), // Orange
matrix.Color(255, 255, 255) // White
};
void setup() {
matrix.begin();
matrix.setTextWrap(false); // Disable text wrapping
matrix.setBrightness(40); // Set brightness (0-255)
}
void loop() {
matrix.fillScreen(0); // Clear the screen
int pos = textPos; // Temporary position for each character
// Loop through each character in the message
for (int i = 0; i < strlen(message); i++) {
// Set the color based on the character's index (mod number of colors)
matrix.setTextColor(colors[i % 8]);
// Print each letter at its corresponding position
matrix.setCursor(pos, 0);
matrix.print(message[i]);
// Move the cursor position to the left by 6 pixels (character width)
pos += 6;
}
matrix.show(); // Refresh the display
delay(100); // Delay for scrolling speed
// Move the text left
textPos--;
// Reset the position when the text has fully scrolled off the left
int16_t textWidth = 6 * strlen(message); // 6 pixels per character for size 1
if (textPos < -textWidth) {
textPos = MATRIX_WIDTH; // Reset position to scroll again
}
}
After uploading this code, you’ll see each letter of your message displayed in different colors as they scroll across the matrix.

Flashing Text with Colorful Letters
For a different effect, you can make each letter flash sequentially with different colors. Here’s the code for that:
#include <Adafruit_GFX.h> // Core graphics library
#include <Adafruit_NeoMatrix.h> // For NeoPixel Matrix
#include <Adafruit_NeoPixel.h> // Basic NeoPixel library
#define DATA_PIN 2 // Use GPIO 2 for the WS2812B Data line
#define MATRIX_WIDTH 8 // Width of the matrix (8x8)
#define MATRIX_HEIGHT 8 // Height of the matrix
// Initialize the matrix with different orientation
Adafruit_NeoMatrix matrix = Adafruit_NeoMatrix(MATRIX_WIDTH, MATRIX_HEIGHT, DATA_PIN,
NEO_MATRIX_TOP + NEO_MATRIX_RIGHT + // Change NEO_MATRIX_LEFT to NEO_MATRIX_RIGHT
NEO_MATRIX_COLUMNS + NEO_MATRIX_PROGRESSIVE,
NEO_GRB + NEO_KHZ800);
// Your flashing message
char message1[] = "Hello World! "; // Add spaces for smoother scrolling
// Define some colors for each letter
uint16_t colors[] = {
matrix.Color(255, 0, 0), // Red
matrix.Color(0, 255, 0), // Green
matrix.Color(0, 0, 255), // Blue
matrix.Color(255, 255, 0), // Yellow
matrix.Color(255, 0, 255), // Magenta
matrix.Color(0, 255, 255), // Cyan
matrix.Color(255, 165, 0), // Orange
matrix.Color(255, 255, 255) // White
};
void setup() {
matrix.begin();
matrix.setTextWrap(false); // Disable text wrapping
matrix.setBrightness(40); // Set brightness (0-255)
}
void loop() {
flashMessage(message1);
}
void flashMessage(char* message) {
int messageLength = strlen(message);
for (int i = 0; i < messageLength; i++) {
matrix.fillScreen(0); // Clear the screen
// Set color for each character
matrix.setTextColor(colors[i % 8]);
// Set cursor position to center the character
matrix.setCursor((MATRIX_WIDTH - 6) / 2, (MATRIX_HEIGHT - 8) / 2);
// Display the current character
matrix.print(message[i]);
matrix.show(); // Update the display
delay(500); // Delay between each flash (adjust for speed)
}
}

By following this tutorial, you’ve created a vibrant, programmable text display using the ESP8266 and WS2812B LED panel. With the flexibility to customize the text, colors, and effects, you can adapt this project for various applications, whether for personal use or to impress your friends and family. Happy coding!