In this guide, we’ll break down the process of displaying both the LAN IP (local IP) and WAN IP (public IP) addresses on the MAX7219 8-Digit 7 Segment Display using the ESP32 Node MCU. Follow these steps carefully, and you’ll be able to showcase your network information on a neat 7-segment display!
Step 1: Gather Materials
Before starting, make sure you have all the necessary materials:
- ESP32 Node MCU
- MAX7219 8-Digit 7 Segment Display
- Jumper wires
Step 2: Wiring Setup
Now, let’s connect the ESP32 Node MCU to the MAX7219 display. Follow these instructions to ensure all components are connected correctly.
- Power Connections:
- ESP32 3.3V Pin to MAX7219 VCC Pin (This powers the MAX7219 display).
- ESP32 GND Pin to MAX7219 GND Pin (Ground connection).
- Data Connections:
- MAX7219 DIN Pin to ESP32 GPIO 23 (Data transfer pin).
- MAX7219 CLK Pin to ESP32 GPIO 18 (Clock synchronization pin).
- MAX7219 CS Pin to ESP32 GPIO 5 (Chip select pin).
Once all the connections are made, your ESP32 and MAX7219 display should be wired up and ready for the next step.
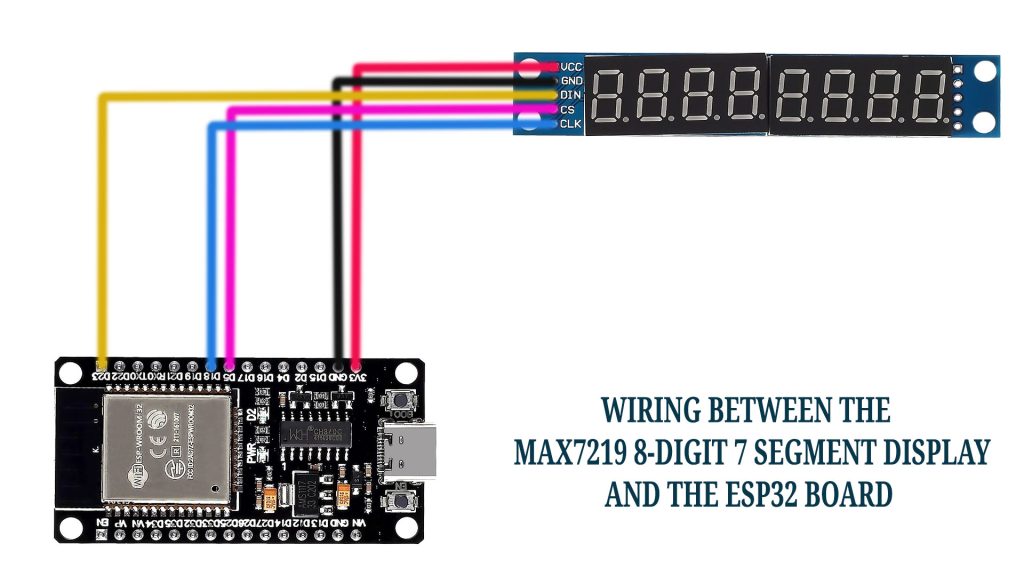
Step 3: Install the LedControl Library
To control the MAX7219 display, you will need the LedControl library in the Arduino IDE. Follow these steps:
- Open Arduino IDE.
- Go to Sketch > Include Library > Manage Libraries.
- In the Library Manager, type “LedControl” in the search bar. The library by Eberhard Fahle should appear in the list. Once you find it, click the “Install” button next to it to add the library to your Arduino IDE. After installation, you can check the “Sketch” > “Include Library” menu to confirm that LedControl has been successfully added to your IDE. This will enable you to start coding for the MAX7219 display with your ESP32.
Note : If you encounter a compilation error like fatal error: avr/pgmspace.h when compiling your code, it’s likely due to compatibility issues with the LedControl library on the ESP32. To fix this, locate the LedControl library folder on your computer. You can usually find this folder in the Arduino/libraries directory. Open the LedControl.cpp or LedControl.h file within that library folder using a text editor. Find the line that includes avr/pgmspace.h (it might look like #include <avr/pgmspace.h>) and comment it out by adding double slashes // at the beginning of the line. This will prevent the code from trying to include that file, as it’s not needed for the ESP32.
Step 4: Set Up Code for LAN IP Display
We will first display the LAN IP (local IP address) of your ESP32 on the MAX7219 8-Digit display.
- Open a new sketch in Arduino IDE.
- Paste the following code for displaying the LAN IP:
#include <WiFi.h>
#include <LedControl.h>
// Your WiFi credentials
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
// Create a LedControl instance (DIN, CLK, CS, number of MAX7219 chips)
LedControl lc = LedControl(23, 18, 5, 1); // DIN to GPIO 23, CLK to GPIO 18, CS to GPIO 5
void setup() {
Serial.begin(115200);
// Connect to WiFi
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
// Initialize the MAX7219
lc.shutdown(0, false); // Wake up the MAX7219
lc.setIntensity(0, 8); // Set brightness level (0-15)
lc.clearDisplay(0); // Clear display register
}
void loop() {
String localIP = WiFi.localIP().toString(); // Get the local IP address
scrollIP(localIP); // Scroll the local IP address
}
// Function to scroll the IP address across the display
void scrollIP(String ip) {
// Pad the IP address with spaces to allow for scrolling
String paddedIP = " " + ip + " "; // Pad with spaces
int length = paddedIP.length();
// Scroll through the padded IP address
for (int i = 0; i < length - 8; i++) { // Stop when the last character is reached
lc.clearDisplay(0); // Clear the display
// Display 8 characters at a time
for (int j = 0; j < 8; j++) {
char c = paddedIP.charAt(i + j); // Get the current character
// Reverse the index for displaying in correct order
int displayIndex = 7 - j;
if (c == '.') {
lc.setChar(0, displayIndex, '.', false); // Display '.' for decimal points
} else if (c >= '0' && c <= '9') {
lc.setDigit(0, displayIndex, c - '0', false); // Display the digit
} else {
lc.setChar(0, displayIndex, ' ', false); // Display a space for other characters
}
}
delay(500); // Wait for a short time before the next scroll
}
}
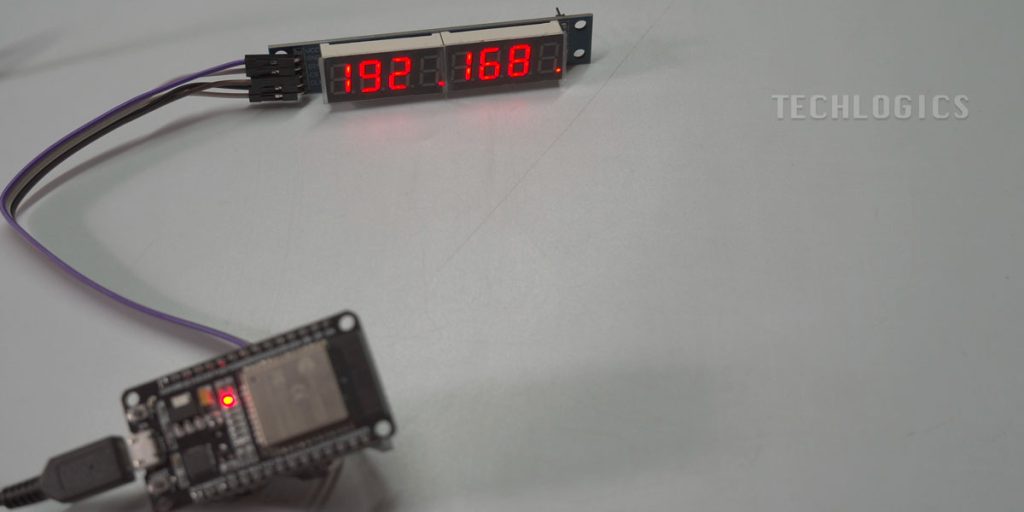
Step 5: Upload Code for LAN IP Display
- In the Arduino IDE, go to Tools > Board and select ESP32 Dev Module (or your specific ESP32 model).
- Go to Tools > Port and select the port corresponding to your ESP32 board.
- Click the Verify button to compile the code.
- Once the code is successfully compiled, click Upload to upload the code to your ESP32 board.
Step 6: View LAN IP Address on MAX7219
After the upload completes, your ESP32 will execute the code, and the LAN IP will be displayed on the MAX7219 8-Digit 7 Segment Display. Additionally, it will also print the LAN IP on the Serial Monitor.
Step 7: Set Up Code for WAN IP Display
Now, let’s set up the code to display the WAN IP (public IP) of your ESP32 on the display. This step will require an external API service to fetch the public IP.
- In the same Arduino sketch, modify the code to retrieve and display the WAN IP:
#include <WiFi.h>
#include <LedControl.h>
#include <HTTPClient.h>
// Your WiFi credentials
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
// Create a LedControl instance (DIN, CLK, CS, number of MAX7219 chips)
LedControl lc = LedControl(23, 18, 5, 1); // DIN to GPIO 23, CLK to GPIO 18, CS to GPIO 5
void setup() {
Serial.begin(115200);
// Connect to WiFi
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.println("Connecting to WiFi...");
}
Serial.println("Connected to WiFi");
// Initialize the MAX7219
lc.shutdown(0, false); // Wake up the MAX7219
lc.setIntensity(0, 8); // Set brightness level (0-15)
lc.clearDisplay(0); // Clear display register
}
void loop() {
String wanIP = getWANIP(); // Get the WAN IP address
scrollIP(wanIP); // Scroll the WAN IP address
}
// Function to get the WAN IP address from an external service
String getWANIP() {
HTTPClient http;
String ip = "";
// Make a GET request to the external IP service
http.begin("http://api.ipify.org"); // IP service URL
int httpCode = http.GET(); // Send the request
if (httpCode > 0) { // Check for the returning code
if (httpCode == HTTP_CODE_OK) { // HTTP 200
ip = http.getString(); // Get the response payload
}
} else {
Serial.printf("Error on HTTP request: %s\n", http.errorToString(httpCode).c_str());
}
http.end(); // Free resources
return ip; // Return the IP address as a string
}
// Function to scroll the IP address across the display
void scrollIP(String ip) {
// Pad the IP address with spaces to allow for scrolling
String paddedIP = " " + ip + " "; // Pad with spaces
int length = paddedIP.length();
// Scroll through the padded IP address
for (int i = 0; i < length - 8; i++) { // Stop when the last character is reached
lc.clearDisplay(0); // Clear the display
// Display 8 characters at a time
for (int j = 0; j < 8; j++) {
char c = paddedIP.charAt(i + j); // Get the current character
// Reverse the index for displaying in correct order
int displayIndex = 7 - j;
if (c == '.') {
lc.setChar(0, displayIndex, '.', false); // Display '.' for decimal points
} else if (c >= '0' && c <= '9') {
lc.setDigit(0, displayIndex, c - '0', false); // Display the digit
} else {
lc.setChar(0, displayIndex, ' ', false); // Display a space for other characters
}
}
delay(500); // Wait for a short time before the next scroll
}
}
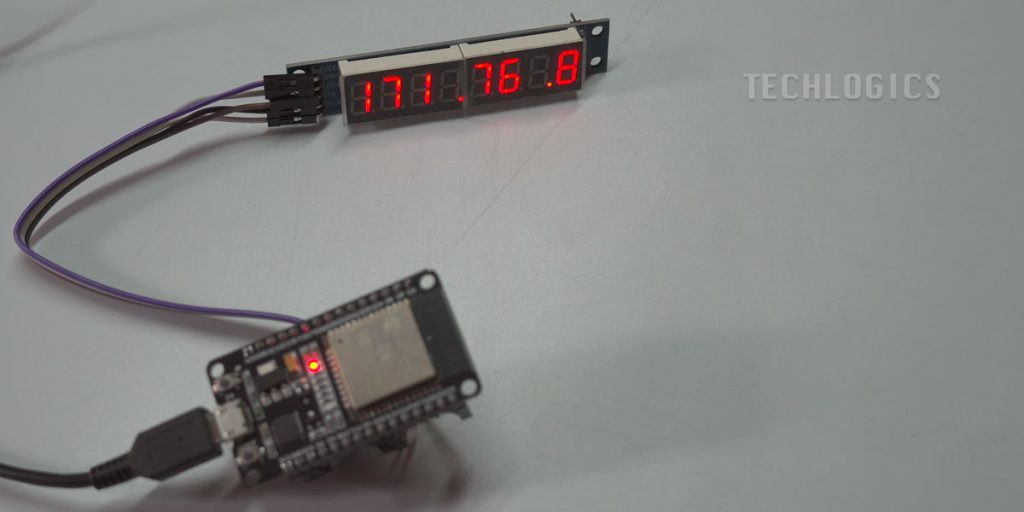
Step 8: Upload Code for WAN IP Display
- After modifying the code, verify and upload the new sketch to the ESP32.
- The WAN IP will now be displayed on the MAX7219 8-Digit 7 Segment Display after a short delay.
Step 9: View WAN IP Address on MAX7219
Once the upload is complete, the WAN IP will appear on the MAX7219 display after 5 seconds, allowing you to easily monitor your public IP address alongside the local one.
Conclusion
You’ve successfully set up the ESP32 to display both the LAN IP and WAN IP on the MAX7219 8-Digit 7 Segment Display. This simple project helps visualize network information and is perfect for monitoring your device’s IP addresses in real-time.